Drone’s Encrypted Flight Logs
This blog post is to act as a basic guide on how to decompile APK’s to locate decryption keys. Specific focus will be on the Yuneec’s flight log data. Some of my research has been reduced to it’s key points, or stripped out entirely where it had lead to a dead end. To follow the quote:
The shortest path is only evident at the end of the journey.
Pre-Requisites
Some basic understanding on mobile phone examination, reverse engineering, and possibly programming is required to perform these actions in your own digital investigations. This is not strictly just for this particular application.
Some basic tools you will need are:
- Flight logs from a ST-16 drone controller: both plaintext and encrypted.
- APK Decompiler, such as jadx.
- Hex editor/viewer, such as HxD.
Obtaining the Flight Logs
The flight logs are stored in the Yuneec ST-16 controller, and can be viewed directly within the File Manager application. You may either transfer the files from the controller to a USB stick using the File Browser, or for a more forensic approach, image the controller using your specialst tool of choice. Most modern tools will detect the controller as an Android based tablet where you may pull a physical extraction.
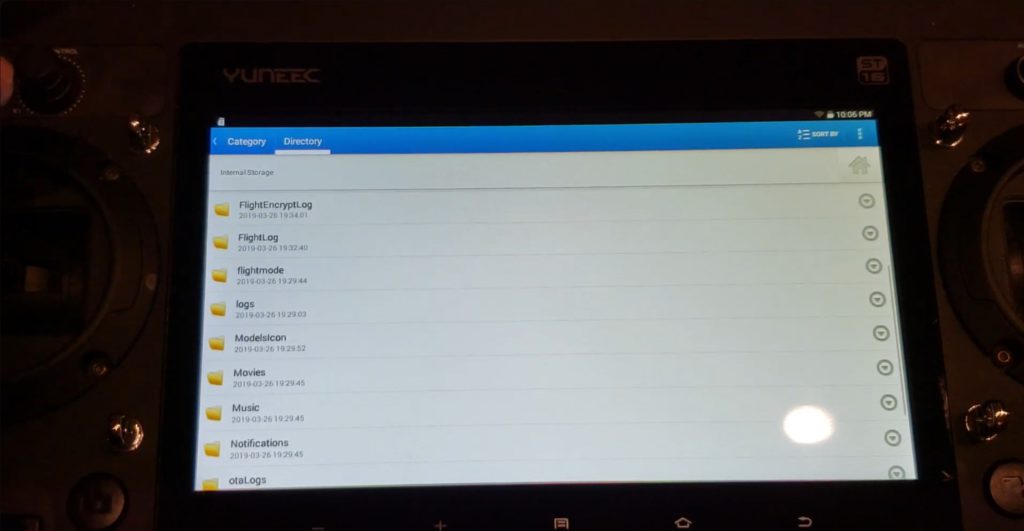
In my case, the flight log files are stored in the following location:
/root/media/0/FlightEncryptLog
/root/media/0/FlightLog
Respectively, the encrypted logs are stored in ‘FlightEncryptLog’, while the plaintext logs are stored in ‘FlightLog’. Each plaintext file has a matching encrypted file. For example:
Plaintext | Encrypted |
/root/media/0/FlightLog/Remote/Remote_00064.c sv | /root/media/0/FlightEncryptLog/Remote/Remote_00064.csv |
/root/media/0/FlightLog/RemoteGPS/RemoteGPS_00016.csv | /root/media/0/FlightEncryptLog/RemoteGPS/RemoteGPS_00016.csv |
/root/media/0/FlightLog/Telemetry/Telemetry_00042.csv | /root/media/0/FlightEncryptLog/Telemetry/Telemetry_00042.csv |
In some situations if the user or system has attempted to delete the logs only the plaintext logs are deleted, while the encrypted logs remain. This can easily be checked by finding encrypted files without a matching plaintext file. It is these encrypted files we are most interested in. Copy these out to a working directory.
If you were to look at the differences between the plaintext files the first line (~200 bytes for ‘Telemetry_00042.csv’) is the CSV header values, and this is consistent amongst all plaintext files where they have an identical header. This similarity is also present amongts the encrypted files, where the first few bytes are always identical. We can determine this is likely a very weak kind of encryption.
Decompiling and Locating the Key
The ST-16 controller uses various Yuneec applications, you will want the ‘FlightMode.apk’, ‘droneFly.apk’, or if you don’t have access to these APK’s from your forensic extraction, then you may use the ‘Yuneec-App-Release.apk’ which was made available by Yuneec staff on the forum here. For this example, i’ll be using the ‘Yuneec-App-Release.apk’.
Launch jadx and import the ‘Yuneec-App-Release.apk’ file to run the decompilation process. In this example, I’m using jadx v1.5.1 with Java 23.0.1. If the decompilation was successfull you should have a screen like this:
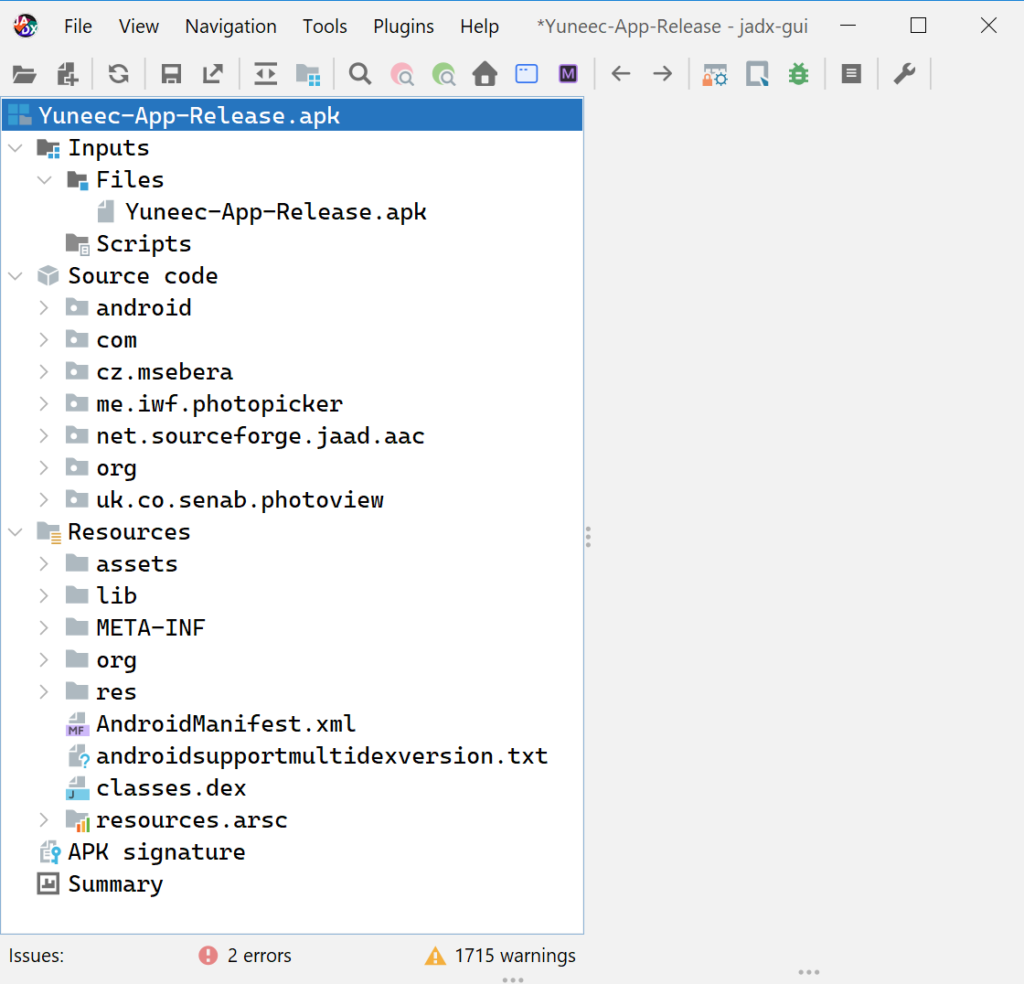
At this point some programming knowledge is ideal to understand the codebase. What we want to look for is any reference to encryption/decryption or even a known value such as the folder name ‘FlightEncryptLog
‘. Jadx has text search built in to run some keywords. Alternatively, you may want to export out the decompiled code base and open it in a text editor/IDE for more powerful features such as tracking/tracing functions calls and indexing for faster searches. In this case we’re going to search for the ‘FlightEncryptLog
‘.
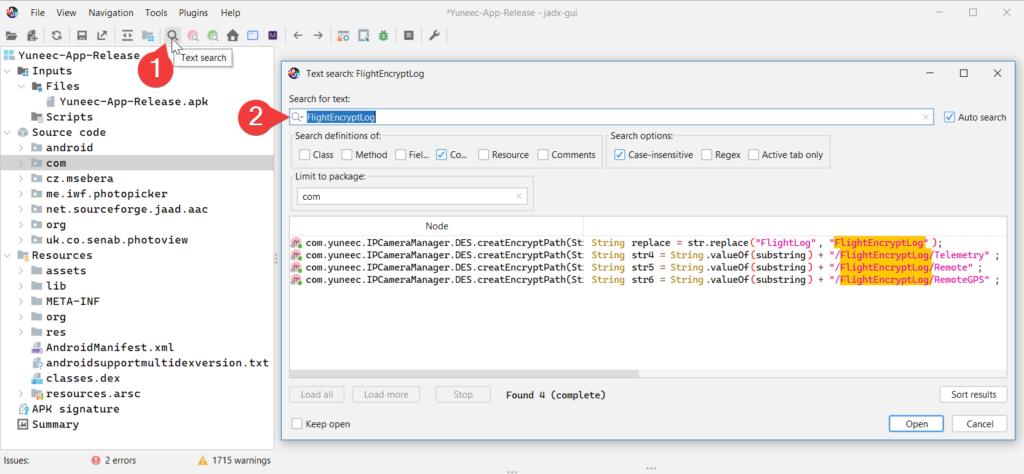
There’s some positive results returned from this search query, all of which come from the class ‘DES
‘ which is found in the decompiled source code folder path ‘/com/yuneec/IPCameraManager
‘. Fortunetly, the search hits land us directly within the class containing the encryption/decryption functions/methods. If you are decompiling an alternative APK file, you may need to search for those functions which is where a powerful IDE may assist.
Looking at the ‘DES
‘ class, if you’re familiar with Java (or a language with a similar syntax) you will find a variable holding a key:
private static final String key = "ksYuN2eC";
Followed by functions named ‘decrypt
‘ and ‘encrypt
‘ which use the DES cipher, and the key above as part of it’s Key and IV arguments:
cipher = Cipher.getInstance("DES/CBC/PKCS5Padding"); cipher.init(2, SecretKeyFactory.getInstance("DES").generateSecret(new DESKeySpec(key.getBytes("UTF-8"))), new IvParameterSpec(key.getBytes("UTF-8")));
With this information we can attempt to decrypt a file using these arguments. As a trial you may use CyberChef with a segment of a plaintext file and compare the results with an encrypted version. A common segment amongst both files as mentioned earlier is the CSV header values. Using CyberChef the plaintext header values were given as an input value:
,fsk_rssi,voltage,current,altitude,latitude,longitude,tas,gps_used,fix_type,satellites_num,roll,yaw,pitch,motor_status,imu_status,press_compass_status,f_mode,gps_status,vehicle_type,error_flags1,gps_accH
Then add in the ‘DES Encrypt’ function using the following arguments:
Key as UTF8: | ksYuN2eC |
IV as UTF8: | ksYuN2eC |
Mode: | CBC |
Input: | Raw |
Output: | Hex |
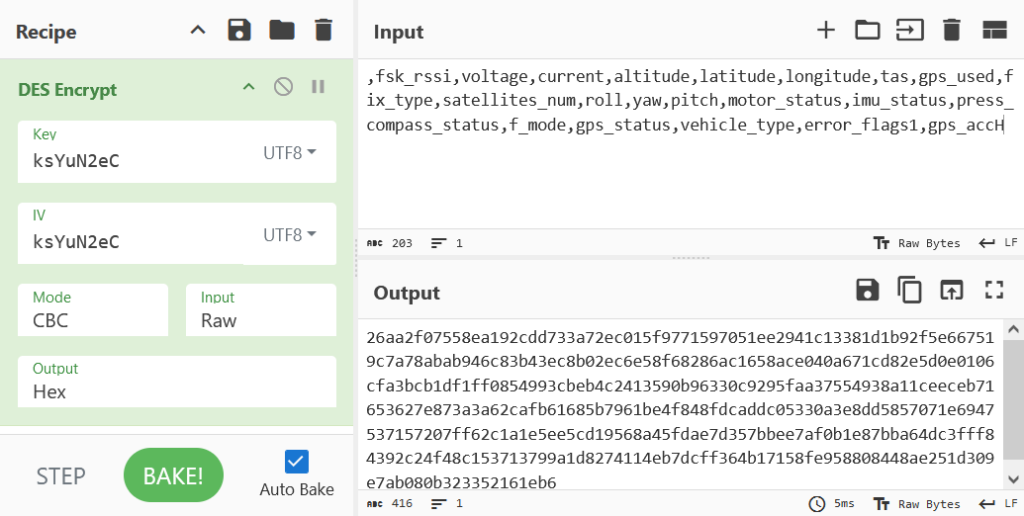
Comparing the HEX values of this encrypted state with an actual encrypted file shows the similarities:
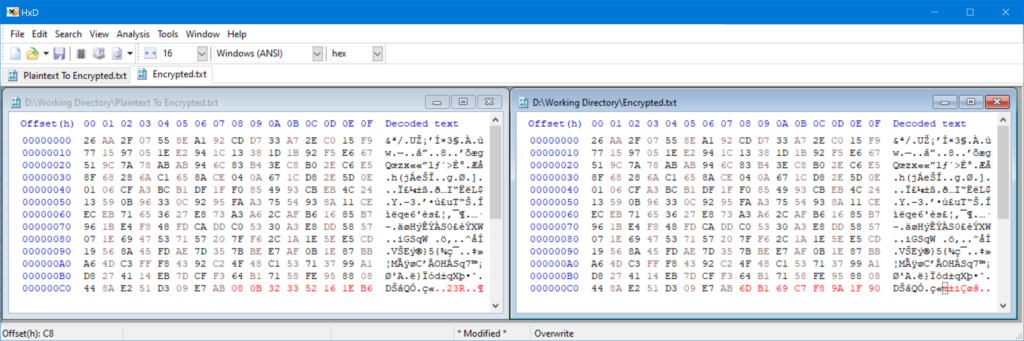
Note the last 16 bytes (highlighted in red) differ due to the padding and additional content within the encrypted file.
In this state it is just a working example of the encryption/decryption process within CyberChef and it is not a feasible option to decrypt many files at once in such a repetative task. Lets move onto decrypting all the files at once.
Programming a Solution
From all of the above information a C# console application was created to assist examiners to decrypt these files in bulk. The current version (at the time of writing) 0.1 is an initial release, with more features to soon follow. The source code is available on GitHub with release builds available for Windows.